Introduction
In this article we will discuss how can we deploy a NodeJS App using google cloud app engine. We will deploy a backend application to the app engine.
Requirements
- Google Cloud Accounts
- Installed NodeJS in your local machine
Preparation
Installing The Cloud SDK
In this article we will be deploying our cloud app engine using google cloud SDK, it is simply a CLI Environment that you could install on your machine to interact with the google cloud services. To install the google cloud SDK you can follow the official documentation here. Assuming that you have installed the cloud sdk we can proceed to the next step.
To make sure that you have google cloud sdk on your computer you could simply open terminal and type
gcloud -v
Make A Project on Google Cloud
If this is the first time you tried google cloud, you need to make a project first. Creating a project is pretty quick and straight forward, the you can follow the steps here.
After you create a project you should be able to select which project work with.
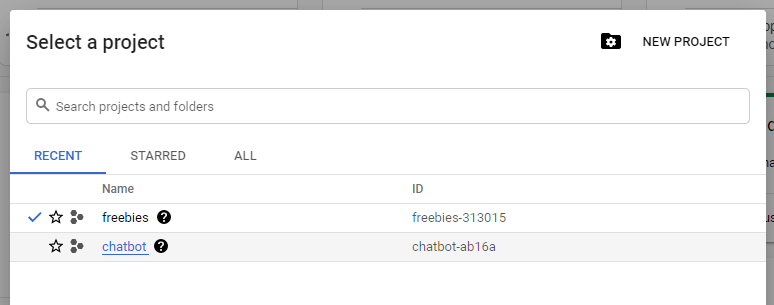
The App
Creating The App
In this article we will just make a simple express with few routes. Create new project folder and do :
npm init --y
npm install express
In the root of your project simply create index.js
, and fill it with
const Express = require('express')
const app = Express();
app.get('/', (req,res) => {
res.send('It is running')
});
const animals = ['Cow', 'Dog', 'Cats']
app.get('/animals', (req,res) => {
res.status(200).send(animals);
})
const PORT = process.env.PORT || 8080;
app.listen(PORT, ()=>{
console.log(`Server is listening on port ${PORT}`)
})
And create a start script on your package.json
"scripts": {
"start" : "node index.js"
},
To run the app you can use
PORT=3030 npm start
The script above will set the process.env.PORT
into 3030. I did this because i have a running xampp server on my local machine. Since it is a GET
request we can simply open it on the browser, when you open /animals
you should get a result like this :
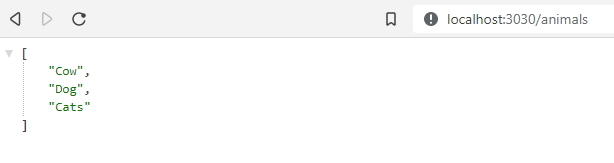
Preparing For Deployment
To make GCP recognize that this project is an App Engine, create a app.yaml
on the root of your projects. There is a lot of options that you can configure such as, what kind of runtime, instance_class, env_variables. here is an example of app.yaml
configuration I used in this article.
runtime: nodejs14
env: standard
instance_class: F1
env_variables:
PORT: "8080"
To make sure that our app engine is running with the GCP free tier checkout : https://cloud.google.com/free/docs/gcp-free-tier
Deploying
After you got your app.yaml
ready, open a terminal in your project root, and do the following :
gcloud init
You will be asked few basic configuration, just make sure that you are using the right account and selecting the right projects. After the initialization is completed, type :
gcloud app deploy
It will take sometimes for it to prepare and deploy your projects to the App Engine. When the process is done you could manually copy the deployment links or type
gcloud app browse
To automatically open your project in the browser. if there is no error your app should be deployed and running!

My Takes
For me it was quite surprising how easy it is to deploy an app to google cloud app engine, note that we are running a single application server here, in some cases when the frontend and backend of the app are in the same codebase this scenario will work just fine, but what if we wanted to separate our frontend and backed?? That topic deserve an article of it’s own lol.
Thankyou for reading this short and unprofessionally written article! Please feel me to ask anything or send me a critique in the comment section down below! See you in the next one!