Introduction
The software utility cron also known as cron job is a time-based job scheduler in Unix-like computer operating systems. Users who set up and maintain software environments use cron to schedule jobs (commands or shell scripts) to run periodically at fixed times, dates, or intervals. It typically automates system maintenance or administration—though its general-purpose nature makes it useful for things like downloading files from the Internet and downloading email at regular intervals. (https://en.wikipedia.org/wiki/Cron)
From definition above we can imagine a lot of application for cron jobs, here are other use case that can be done using cron job :
- Scheduling Push Notification
- Create weekly email notification
- Schedule cache cleaning
- Schedule database backups
- Schedule user rotation
And a lot more, for application 3 & 4 yes for sure that you could do that using cron jobs, but usually there are better ways to implement database backups or cache expiration.
Implementation
In this article we will create a basic implementation of cron job using node-cron.
Creating Projects & Installing Dependencies
Create a new folder for the project
$ mkdir cron-job-nodejs
In the project root, initialize npm. This command will initialize npm on the project root and setting all configuration as default.
$ npm init --y
Then install the dependencies. express will be used as the webserver and node-cron will act as the scheduler.
$ npm install express node-cron
Setting up Web Server
Let’s create a minimal web server, first create index.js
in the root project folder.
// index.js
const express = require('express');
const cron = require('node-cron');
const app = express();
app.listen(3000, ()=>{
console.log(`App listening on port 3000`)
})
Adding the cron job.
// index.js
const app = express();
cron.schedule('* * * * *', () => {
console.log('running a task every minute');
});
app.listen(3000, ()=>{
console.log(`App listening on port 3000`)
})
You try to wait for a couple minutes and you should see this output :
$ node index.js
App listening on port 3000
running a task every minute
running a task every minute
running a task every minute
running a task every minute
running a task every minute
running a task every minute
The asterisks are part of the node-cron syntax to represent different units of time :
# ┌────────────── second (optional)
# │ ┌──────────── minute
# │ │ ┌────────── hour
# │ │ │ ┌──────── day of month
# │ │ │ │ ┌────── month
# │ │ │ │ │ ┌──── day of week
# │ │ │ │ │ │
# │ │ │ │ │ │
# * * * * * *
Taken from : https://www.npmjs.com/package/node-cron
Here is an example format to run a command for every second,
cron.schedule('*/1 * * * * *', () => {
console.log('running a task every seconds');
});
Email Cron Job
First we need to install nodemailer
$ npm install nodemailer
Create a mail.js
file
$ touch mail.js
For this project i am using mailtrap as the smtp server, it is a great tool for development. it provides you with an dev only smtp server that is free to use. read more about it here https://mailtrap.io/. If you want to follow along with the tutorials you could register there first, but if you wanted to use other smtp it is also good. Here is my mail.js
looks like.
const nodemailer = require('nodemailer');
async function sendMail(){
try{
var transport = nodemailer.createTransport({
host: "smtp.mailtrap.io",
port: 2525,
auth: {
user: "<<user from mailtrap>>",
pass: "<<passwor from mailtrap>>"
}
});
const mailOptions = {
from : 'Arif Luthfi 😯 <arifluthfi16@gmail.com>',
to : 'arifluthfi16.gtest@gmail.com',
subject : 'Email Scheduler',
text : 'Hello from spambot',
html : '<h1>Email scheduler</h1>'
}
const result = await transport.sendMail(mailOptions);
return result
}catch(error){
return error
}
}
module.exports = sendMail;
Then modify index.js
cron scheduler to run your mailer
const express = require('express');
const cron = require('node-cron');
const sendMail = require('./mail');
const app = express();
cron.schedule('*/3 * * * *', () => {
console.log("Sending Email Every 3 Minutes")
sendMail();
});
app.listen(3000, ()=>{
console.log(`App listening on port 3000`)
})
Run the app and wait for 3 minutes, if you also use mailtrap, you should get an email like this.
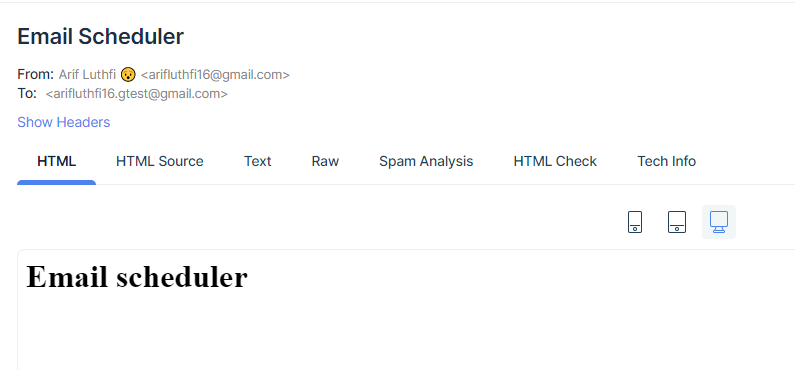
That’s all! Thanks for reading I hope this article could help anyone who needs it. feel free to ask question in comment section!
Source Code
You can get the source code here